Development
Automatic Deployment with GitHub on Any Server Instance without GitHub workflow or SSH
Learn to impliment automatic deployment an application to the cloud virtual server without using GitHub workflow and SHH, by means of a nodejs based express server.
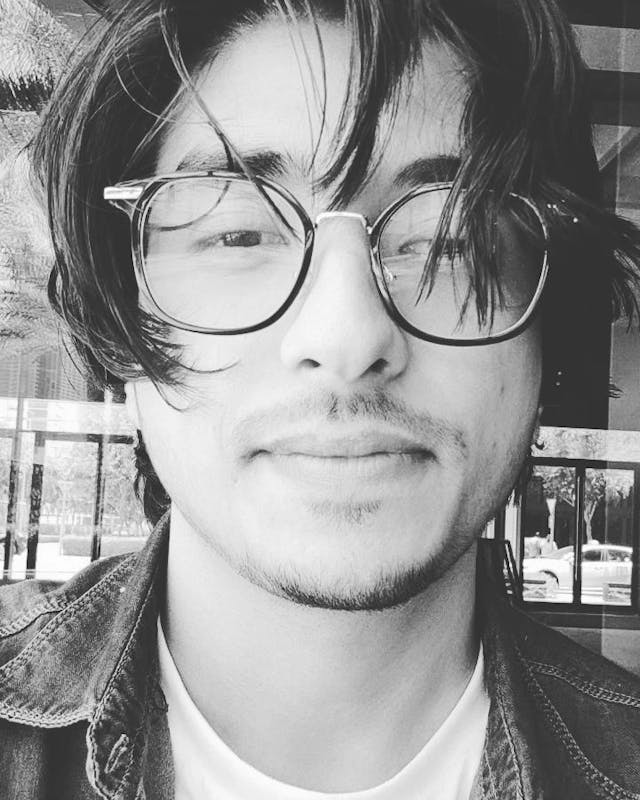
Raja Osama
I Describe Myself as a Polyglot ~ Tech Agnostic ~ Rockstar Software Engineer. I Specialise in Javascript-based tech stack to create fascinating applications.
I am also open for freelance work, and in-case you want to hire me, you can contact me at rajaosama.me@gmail.com or contact@rajaosama.me